極座標変換(原点からの角度)
Namespace: FVIL.CaliperAssembly: FVILbasic (in FVILbasic.dll) Version: 3.1.0.0 (3.1.0.17)
Syntax
C# |
---|
public static CFviAngle PolarCoordinatesAngle(
CFviPoint point
) |
Visual Basic |
---|
Public Shared Function PolarCoordinatesAngle (
point As CFviPoint
) As CFviAngle |
Return Value
Type:
CFviAngle
指定点(
P)と原点の角度(θ)を求めます。
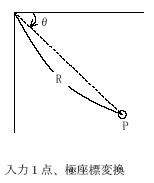
正常に実行できなかった場合は例外を発行します。
例外の原因と発生位置を特定するには、発行された例外クラスの ErrorCode メンバと Function メンバを参照してください。
エラーコード:
値 | ErrorCode メンバ | 内容 |
---|
51 | FVIL.ErrorCode.LICENSE_ERROR |
ライセンスキーが見つからない為、実行できません。
または、 FVIL._SetUp.InitVisionLibrary が実行されていません。
|
11 | FVIL.ErrorCode.INVALID_PARAMETER | パラメータに誤りがあります。 |
Examples
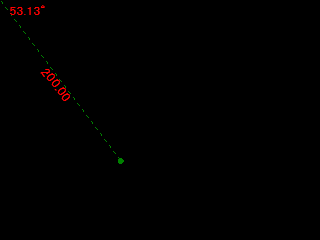
C# | Copy |
---|
using System;
using System.Collections.Generic;
using System.Text;
using System.Drawing;
using fvalgcli;
namespace User.SampleCode
{
public partial class Caliper
{
[FvPluginExecute]
public void PolarCoordinatesAngle()
{
FVIL.Data.CFviPoint point = new FVIL.Data.CFviPoint(120,160);
FVIL.Data.CFviAngle angle = FVIL.Caliper.Function.PolarCoordinatesAngle(point);
double distance = FVIL.Caliper.Function.PolarCoordinatesDistance(point);
{
FVIL.Data.CFviImage image = new FVIL.Data.CFviImage(320, 240, FVIL.ImageType.UC8, 1);
FVIL.GDI.CFviDisplay display = new FVIL.GDI.CFviDisplay();
FVIL.GDI.CFviOverlay overlay = new FVIL.GDI.CFviOverlay();
{
FVIL.GDI.CFviGdiPoint gpoint1 = new FVIL.GDI.CFviGdiPoint(point);
gpoint1.Pen.Color = Color.Green;
gpoint1.Pen.Width = 4;
FVIL.Data.CFviLineSegment lineseg = new FVIL.Data.CFviLineSegment(new FVIL.Data.CFviPoint(0,0), point);
FVIL.GDI.CFviGdiLineSegment gline1 = new FVIL.GDI.CFviGdiLineSegment(lineseg);
gline1.Pen.Color = Color.Green;
gline1.Pen.Width = 1;
gline1.Pen.Style = FVIL.GDI.PenStyle.Dot;
FVIL.Data.CFviPoint pos = new FVIL.Data.CFviPoint();
string str_angle = String.Format("{0:F}°", angle.Degree);
FVIL.GDI.CFviGdiString gStrAngle = new FVIL.GDI.CFviGdiString(str_angle);
pos.X = 8;
pos.Y = 4;
gStrAngle.Position = pos;
gStrAngle.Font.Weight = FVIL.GDI.FontWeight.Thin;
gStrAngle.Font.Height = 12;
gStrAngle.Font.Width = 6;
gStrAngle.Color = Color.Red;
string str_dist = String.Format("{0:F}", (int)(distance));
FVIL.GDI.CFviGdiString gStrDist = new FVIL.GDI.CFviGdiString(str_dist);
gStrDist.Position = FVIL.Caliper.Function.CenterPoint(new FVIL.Data.CFviPoint(0, 0), point);
gStrDist.Align = FVIL.GDI.TextAlign.Center;
gStrDist.Angle = angle;
gStrDist.Font.Weight = FVIL.GDI.FontWeight.Thin;
gStrDist.Font.Height = 12;
gStrDist.Font.Width = 6;
gStrDist.Color = Color.Red;
overlay.Figures.Add(gpoint1);
overlay.Figures.Add(gline1);
overlay.Figures.Add(gStrAngle);
overlay.Figures.Add(gStrDist);
overlay.Enable = true;
}
display.Overlays.Add(overlay);
display.Image = image;
display.DisplayRect = image.Window;
FVIL.Data.CFviImage dstimage = new FVIL.Data.CFviImage();
display.SaveImage(dstimage);
FVIL.File.Function.SaveImageFile(Defs.ResultDir + "/Caliper.PolarCoordinatesAngleDistance.png", dstimage);
}
}
}
} |
Visual Basic | Copy |
---|
Imports System.Collections.Generic
Imports System.Text
Imports System.Drawing
Imports fvalgcli
Namespace SampleCode
Public Partial Class Caliper
<FvPluginExecute> _
Public Sub PolarCoordinatesAngle()
Dim point As New FVIL.Data.CFviPoint(120, 160)
Dim angle As FVIL.Data.CFviAngle = FVIL.Caliper.[Function].PolarCoordinatesAngle(point)
Dim distance As Double = FVIL.Caliper.[Function].PolarCoordinatesDistance(point)
If True Then
Dim image As New FVIL.Data.CFviImage(320, 240, FVIL.ImageType.UC8, 1)
Dim display As New FVIL.GDI.CFviDisplay()
Dim overlay As New FVIL.GDI.CFviOverlay()
If True Then
Dim gpoint1 As New FVIL.GDI.CFviGdiPoint(point)
gpoint1.Pen.Color = Color.Green
gpoint1.Pen.Width = 4
Dim lineseg As New FVIL.Data.CFviLineSegment(New FVIL.Data.CFviPoint(0, 0), point)
Dim gline1 As New FVIL.GDI.CFviGdiLineSegment(lineseg)
gline1.Pen.Color = Color.Green
gline1.Pen.Width = 1
gline1.Pen.Style = FVIL.GDI.PenStyle.Dot
Dim pos As New FVIL.Data.CFviPoint()
Dim str_angle As String = [String].Format("{0:F}°", angle.Degree)
Dim gStrAngle As New FVIL.GDI.CFviGdiString(str_angle)
pos.X = 8
pos.Y = 4
gStrAngle.Position = pos
gStrAngle.Font.Weight = FVIL.GDI.FontWeight.Thin
gStrAngle.Font.Height = 12
gStrAngle.Font.Width = 6
gStrAngle.Color = Color.Red
Dim str_dist As String = [String].Format("{0:F}", CInt(Math.Truncate(distance)))
Dim gStrDist As New FVIL.GDI.CFviGdiString(str_dist)
gStrDist.Position = FVIL.Caliper.[Function].CenterPoint(New FVIL.Data.CFviPoint(0, 0), point)
gStrDist.Align = FVIL.GDI.TextAlign.Center
gStrDist.Angle = angle
gStrDist.Font.Weight = FVIL.GDI.FontWeight.Thin
gStrDist.Font.Height = 12
gStrDist.Font.Width = 6
gStrDist.Color = Color.Red
overlay.Figures.Add(gpoint1)
overlay.Figures.Add(gline1)
overlay.Figures.Add(gStrAngle)
overlay.Figures.Add(gStrDist)
overlay.Enable = True
End If
display.Overlays.Add(overlay)
display.Image = image
display.DisplayRect = image.Window
Dim dstimage As New FVIL.Data.CFviImage()
display.SaveImage(dstimage)
FVIL.File.[Function].SaveImageFile(Defs.ResultDir & "/Caliper.PolarCoordinatesAngleDistance.png", dstimage)
End If
End Sub
End Class
End Namespace |
Exceptions
See Also